Or quick tips on how to implement your own inputLookup Salesforce ligthning component
This is a repost of the article I published on Salesforce Developer Blog.
Salesforce Spring '15 release brought some brand new components ready to be used in your lightning apps. One of the missing components that could be useful for your apps is the input lookup component. The idea is to use a typeahead input field. We are going to user Bootstrap and the Twitter typeahead component.
The idea behind this implementation is that the user will type a search on the input field and the typeahead shows a list of the first 20 items for the given object and search string. On load, the component should also load the initial value of the "name" field for the given object, if an ID value is selected.
This is how the component will look:
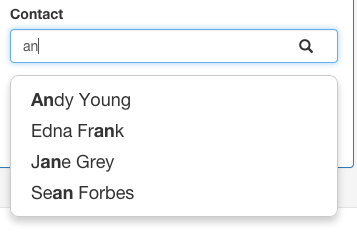
For those of you who don't want to read more and just use the component, jump to the inputLookup github repo.
To ensure proper script loading we will use RequireJS with the trick proposed in this blog post.
Let's first have a look at the lookupInput component's markup (github):
<aura:component controller="InputLookupController"> <ltng:require scripts="/resource/RequireJS" afterScriptsLoaded="{!c.initTypeahead}" /> <aura:handler name="init" value="{!this}" action="{!c.setup}"/> <!-- ... --> <!-- public attributes --> <aura:attribute name="type" type="String" required="true"/> <aura:attribute name="value" type="String" /> <aura:attribute name="className" type="String" /> <!-- PRIVATE ATTRS --> <aura:attribute name="nameValue" type="String" access="PRIVATE"/> <aura:attribute name="isLoading" type="Boolean" default="true" access="PRIVATE"/> <div class="has-feedback"> <input id="{!globalId+'_typeahead'}" type="text" value="{!v.nameValue}" class="{!v.className}" onchange="{!c.checkNullValue}" readonly="{!v.isLoading}"/> <span class="glyphicon glyphicon-search form-control-feedback"></span> </div> </aura:component>It uses the "InputLookupController" Apex class (with required @RemoteActions).
The RequireJS components triggers "c:requireJSLoaded" event that actually makes the component load its bootstrapped Typeahead input field: note the absence of any custom namespace, that is replaced with the "c" default namespace (this is a Spring '15 feature).
We also have an init method that ensures that all required parameters are set.
This component simply uses a "type" parameters (with the actual API name of the needed SObject) and a "value" parameter that stores the selected ID (or the loading ID).
At first we want to load on initialization (or when the value parameter changes) the content of the input (we want to see the "Name" of the pre-selected SObject and not its ID).
The initialization loading is done using the following "inputLookupController.js" method:
initTypeahead : function(component, event, helper){ try{ //first load the current value of the lookup field and then //creates the typeahead component helper.loadFirstValue(component); }catch(ex){ console.log(ex); } }This is the helper part:
loadFirstValue : function(component) { //this is necessary to avoid multiple initializations (same event fired again and again) if(this.typeaheadInitStatus[component.getGlobalId()]){ return; } this.typeaheadInitStatus[component.getGlobalId()] = true; this.loadValue(component); }, loadValue : function(component, skipTypeaheadLoading){ this.typeaheadOldValue[component.getGlobalId()] = component.get('v.value'); var action = component.get("c.getCurrentValue"); var self = this; action.setParams({ 'type' : component.get('v.type'), 'value' : component.get('v.value'), }); action.setCallback(this, function(a) { if(a.error && a.error.length){ return $A.error('Unexpected error: '+a.error[0].message); } var result = a.getReturnValue(); component.set('v.isLoading',false); component.set('v.nameValue',result); if(!skipTypeaheadLoading) self.createTypeaheadComponent(component); }); $A.enqueueAction(action); }This piece of code simply calls the "InputLookupController.getCurrentValue" remote action that simply preload the selected record's Name field (if any).
The "typeaheadInitStatus" property (see the full file code) is used to avoid multiple initialization of the component (the "createTypeaheadComponent" method is the method that is used to create the typeahead component: this must be executed only once!).
On the other side the "typeaheadOldValue" stores the current value, in order to understand if the value parameter is changed: this is extremely useful when some other part of the application changes the value parameters and so the component should refresh it's inner value.
To achieve this simply see the "inputLookupRenderer.js" file:
rerender : function(component, helper){ this.superRerender(); //if value changes, triggers the loading method if(helper.typeaheadOldValue[component.getGlobalId()] !== component.get('v.value')){ helper.loadValue(component,true); } }This method is called every time the component is rerendered (e.g. when one of its parameters changes), and it constantly evaluates if the "value" parameter has changed since the last set; if this is true then we load again the component, without re-contructing it ("skipTypeaheadLoading" parameters of the loadValue function).
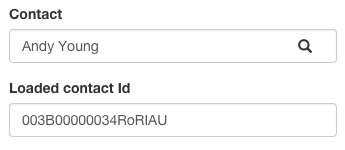
The typeahead is a simple porting of the Twitter Typeahead component with some changes, the most important of which is the call to the remote action that search (using SOSL) for all SObjects given the search term ("substringMatcher" function of the helper file):
// . . . substringMatcher : function(component) { //usefull to escape chars for regexp calculation function escapeRegExp(str) { return str.replace(/[\-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, "\\$&"); } return function findMatches(q, cb) { q = escapeRegExp(q); var action = component.get("c.searchSObject"); var self = this; action.setParams({ 'type' : component.get('v.type'), 'searchString' : q, }); action.setCallback(this, function(a) { if(a.error && a.error.length){ return $A.error('Unexpected error: '+a.error[0].message); } var result = a.getReturnValue(); var matches, substrRegex; // an array that will be populated with substring matches var matches = []; // regex used to determine if a string contains the substring `q` var substrRegex = new RegExp(q, 'i'); var strs = JSON.parse(result); // iterate through the pool of strings and for any string that // contains the substring `q`, add it to the `matches` array $.each(strs, function(i, str) { if (substrRegex.test(str.value)) { // the typeahead jQuery plugin expects suggestions to a // JavaScript object, refer to typeahead docs for more info matches.push({ value: str.value , id: str.id}); } }); if(!strs || !strs.length){ $A.run(function(){ component.set('v.value', null); }); } cb(matches); }); $A.run(function(){ $A.enqueueAction(action); }); }; } // . . .This "complex" code is used to push the results from the "searchSObject" remote action to the typeahead result set picklist.
Finally you can add the component in your lightning app:
<aura:application > <aura:attribute name="id" type="String" default="" access="GLOBAL"/> <aura:attribute name="objNew" type="Contact" default="{'sobjectType':'Contact', 'Id':null}" /> <!-- ... --> <div class="well"> <div class="panel panel-primary"> <div class="panel-heading">Existent sobject</div> <div class="panel-body"> <div class="form-horizontal" > <div class="form-group"> <label class="col-sm-2 control-label">Contact</label> <div class="col-sm-8"> <c:inputLookup type="Contact" value="{!v.id}" className="form-control "/> </div> </div> <div class="form-group has-feedback"> <label class="col-sm-2 control-label">Loaded contact</label> <div class="col-sm-8 "> <ui:inputText value="{!v.id}" class="form-control" placeholder="Change id value"/> </div> </div> </div> </div> </div> <div class="panel panel-primary"> <div class="panel-heading">New sobject</div> <div class="panel-body"> <div class="form-horizontal" > <div class="form-group"> <label class="col-sm-2 control-label">Contact</label> <div class="col-sm-8"> <c:inputLookup type="{!v.objNew.sobjectType}" value="{!v.objNew.Id}" className="form-control "/> </div> </div> <div class="form-group has-feedback"> <label class="col-sm-2 control-label">New contact</label> <div class="col-sm-8 "> <ui:inputText value="{!v.objNew.Id}" class="form-control" placeholder="Change id value"/> </div> </div> </div> </div> </div> </div> </aura:application>We have two components, the first one 's value is grabbed directly from the "?id=XXXX" query string parameters, while the second one has no value on load:
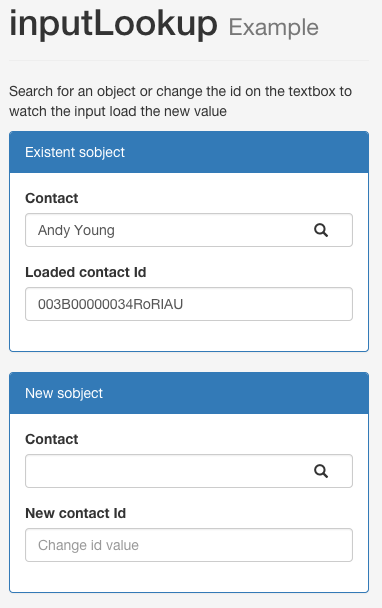
Finally you can easily test that everytime you change the "value" input text, the inputLookup component loads it's new "name" field, while if you search for a Contact on the component and select a record, the value input is set with the new value.
You brand new custom input lookup component is served!
No comments:
Post a Comment